factorial of n ( denoted by n! ) is the product of all positive integers less than or equal to n. For example ,
5! = 5 * 4 * 3 * 2 * 1 = 120
4! = 4 * 3 * 2 * 1 = 24
3! = 3 * 2 * 1 = 6
2! = 2 * 1 = 2
1! = 1 = 1
C program to find the factorial without recursion
#include<stdio.h>
void main()
{
int i,n,fact=1;
clrscr();
printf("Enter number to find out factorial:");
scanf("%d",&n);
for(i=n;i>=1;i--)
{
fact=fact*i;
}
printf("\nFactorial of %d is:: %d",n,fact);
getch();
}
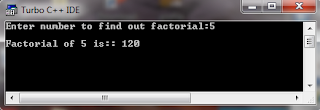
Recursion means a function is call by itself. Best example of recursion is factorial. Consider the following example of factorial by using recursion.
C program to finc factorial using recursion
#include<stdio.h>
void main()
{
int no,ans;
int factorial(int);
clrscr();
printf("\nEnter no to find out factorial: ");
scanf("%d",&no);
ans=factorial(no);
printf("\nfactorial of %d is: %d",no,ans);
getch();
}
int factorial(int x)
{
int fact;
if(x==1)
{
return(1);
}
else
{
fact=x*factorial(x-1);
}
return(fact);
}
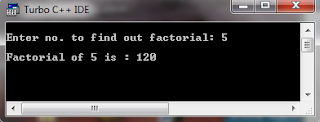
0 comments:
Post a Comment
Click to see the code!
To insert emoticon you must added at least one space before the code.