A program to print triangle or pattern is focus on loop section of the programming. This type of program is for better understanding of concept of looping in programming language. Most of these type of programs need to use the nested loops and space. For example you need to print following triangle.
*
***
*****
*******
*********
This type of triangle need to use for loop in program. They also need some nested loops(loop in a loop is called nested loop). The outer for loop is for defining the rows of the triangle and inner for loop is for the number of character or number or space in each rows. Just see the source code of above triangle so will be get better idea about the loop.
If you want source code of any other pattern just write down your pattern in comment we will provide source code of that pattern.
#include<stdio.h>
void main()
{
int i,j,k,n;
clrscr();
printf("Enter number of row to print triangle::");
scanf("%d",&n);
for(i=1;i<=n;i++)
{
for(k=i;k<=n;k++)
{
printf(" ");
}
for(j=1;j<=2*i-1;j++)
{
printf("*");
}
printf("\n");
}
getch();
}
Now lets consider another half triangle pattern program.
*
**
***
****
*****
#include<stdio.h>
void main()
{
int i,j,k,n;
clrscr();
printf("Enter number of rows:");
scanf("%d",&n);
for(i=1;i<=n;i++)
{
for(k=i;k<=n;k++)
{
printf(" ");
}
for(j=i;j>=1;j--)
{
printf("*");
}
printf("\n");
}
getch();
}
Now lets consider one another example.
*
**
***
****
*****
#include<stdio.h>
void main()
{
int i,j,n;
clrscr();
printf("Enter number of rows:");
scanf("%d",&n);
for(i=1;i<=n;i++)
{
for(j=1;j<=i;j++)
{
printf("*");
}
printf("\n");
}
getch();
}
*
***
*****
*******
*********
This type of triangle need to use for loop in program. They also need some nested loops(loop in a loop is called nested loop). The outer for loop is for defining the rows of the triangle and inner for loop is for the number of character or number or space in each rows. Just see the source code of above triangle so will be get better idea about the loop.
If you want source code of any other pattern just write down your pattern in comment we will provide source code of that pattern.
#include<stdio.h>
void main()
{
int i,j,k,n;
clrscr();
printf("Enter number of row to print triangle::");
scanf("%d",&n);
for(i=1;i<=n;i++)
{
for(k=i;k<=n;k++)
{
printf(" ");
}
for(j=1;j<=2*i-1;j++)
{
printf("*");
}
printf("\n");
}
getch();
}
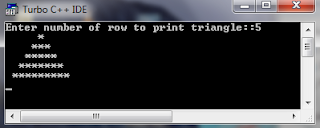
Now lets consider another half triangle pattern program.
*
**
***
****
*****
#include<stdio.h>
void main()
{
int i,j,k,n;
clrscr();
printf("Enter number of rows:");
scanf("%d",&n);
for(i=1;i<=n;i++)
{
for(k=i;k<=n;k++)
{
printf(" ");
}
for(j=i;j>=1;j--)
{
printf("*");
}
printf("\n");
}
getch();
}

Now lets consider one another example.
*
**
***
****
*****
#include<stdio.h>
void main()
{
int i,j,n;
clrscr();
printf("Enter number of rows:");
scanf("%d",&n);
for(i=1;i<=n;i++)
{
for(j=1;j<=i;j++)
{
printf("*");
}
printf("\n");
}
getch();
}
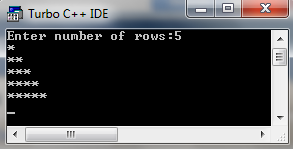
Download |
---|
I need source code for following triangle
ReplyDelete1
2 3
4 5 6
7 8 9 10
i want program for this code
Delete#include
ReplyDelete//#include
void main()
{
int i,j,k;
int count=0;
for(i=0;i<5;i++)
{
for(j=1;j<=i;j++)
{
++count;
printf("%d",count);
}
printf("\n");
}
}
i need the source code of
ReplyDelete1===1===1
=2==2==2=
==3=3=3==
===444===
123454321
===444===
==3=3=3==
=2==2==2=
1===1===1
= means white space...... and this is for number 9
Hi Arun CM here is your solution
Deletehttp://www.programmingcampus.com/2013/07/user-solution-306.html
Sue to some technical problem we write your source code on above page.
********
ReplyDelete*
********
*
*
********
plz give me the code for above pattern